Recently I have decided to write a new expense tracking application to replace the previous quick and dirty one I wrote long ago that I have been using to log my expenses.
The previous version was written using PHP and MySQL and was deployed using Docker on my personal home server. I got lazy to update it with new features as it was quite tedious, so I decided to write a new one using new technologies, also as a way to increase my portfolio of software products.
It is also a way to practice and make use of the new knowledge I acquired from reading books like Unit Testing, Clean Code, Test Driven Development and Refactoring. I also try to use as much knowledge I acquired from working in Optimizely.
The new expense tracking program is called “DotNetExpenseManager”, and will be developed with the following in mind:
- Hosted on Github at https://github.com/brucengdev/DotNetExpenseManager .
- The backend is written using C# and ASP.NET, it serves as a REST API backend for the frontend. xUnit is used for automated tests.
- The backend uses Entity Framework and SQL server to store user data. When deployed on Linux or Docker, I’m using an Azure SQL Server Docker container.
- Database migrations and updates are completely handled using EF Core migration by code.
- The frontend is written using Vite and React in Typescript, using Vitest for automated tests with React-testing-library.
- Fully TDD (Test Driven Development), for most of the time tests are written first before new code is written.
- Apply knowledge I learned from the book Unit Testing principles.
- The project must have Continuous Integration and Continuous Delivery implemented using Github actions and Github workflows.
- Deployment is easy by using Docker and a docker-compose file. Deployment must be automated, so that I don’t spend much time doing deployment. I can just merge new code to the main branch, and everything is automatically deployed.
After few days of working on it, I managed to get the following functions working
- Login/logout as an admin user.
- Log a new expense entry
The UI is super nice, that means it looks absolutely barren 😀 . Because at the moment I’m focusing on implementing the functions first before implementing the UI.

Once login we have a very barren view of your current expenses for today 😀 .
You can log a new entry, but you can’t view your entries, because it has not been implemented xD .
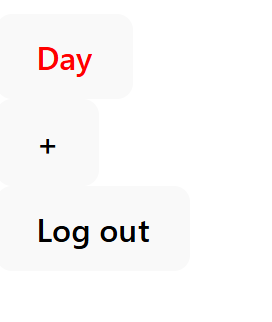
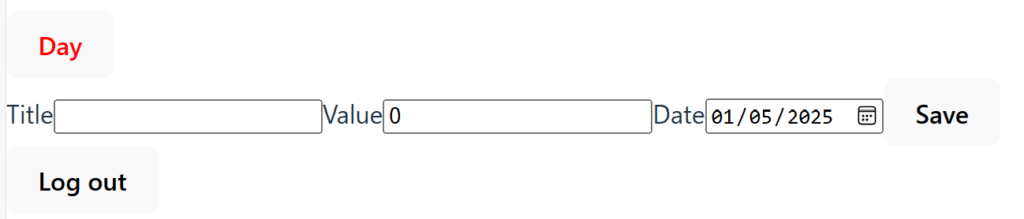
For testing, the ideas are from the book Unit Testing Principles, Practices, and Patterns (Unit Testing Principles, Practices, and Patterns). The idea is
- Mock the input and the output of the system, make sure that the implementation of those layers that glue external systems with the functional core is as straight forward as possible, so that they require little to no testing.
- Try to isolate the functional part of the system as much as possible.
- Test the functional core of the application as much as possible.
So for frontend, the localStorage and http client are mocked using real doubles. The doubles are real Javascript objects that run fast.
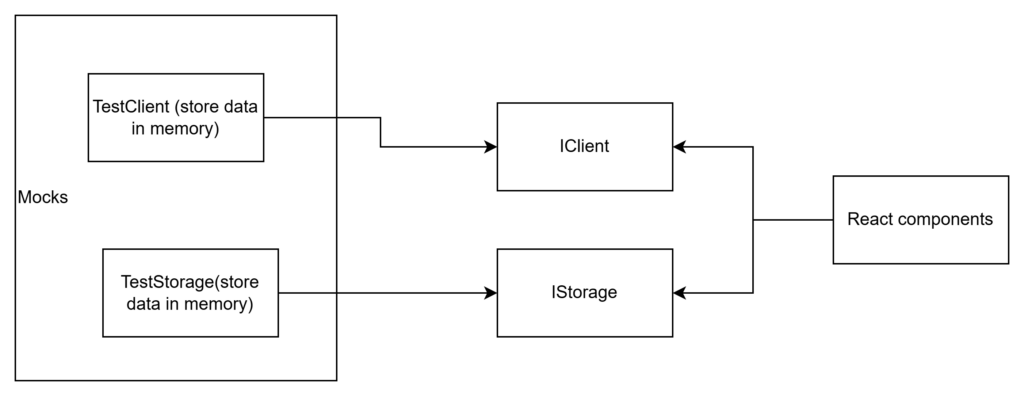
When the app runs, real implementations are used that uses Local Storage for IStorage and fetch APIs for http requests. This implementation makes it easier to change the underlying implementation easily.
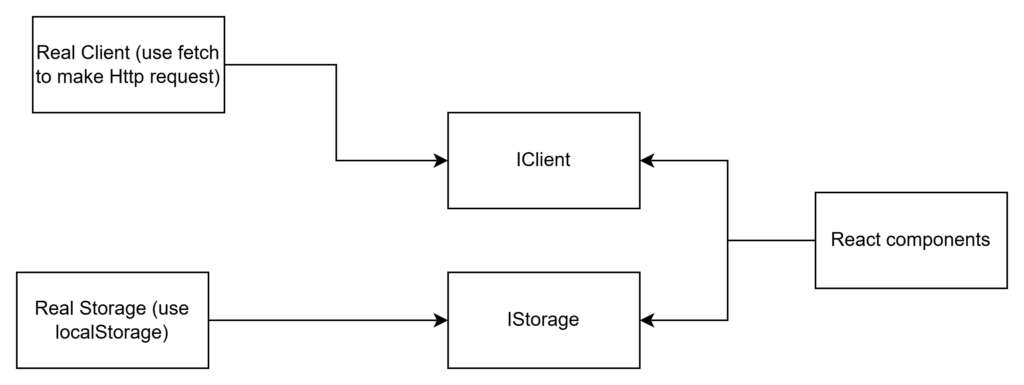
So for example, in the future, the storage may not use localstorage, but using other ways of persistence, or you can totally switch to use other kinds of API for IClient (instead of REST, use Grpc, or GraphQL).
In the backend side, a similar design is used. The main logic (the functional core) is isolated into manager classes like AccountManager and EntryManager. The interface for clients which are the REST controllers is a thin layer that does only trivial tasks.
Similarly, the persistence layer which is the database are encapsulated into repositories like IUserRepository and IEntryRepository. The real implementation uses EntityFramework Database context, but these details are hidden away from the functional core (the managers). The repositories are created to be as straight forward as possible, so that testing it is trivial or not needed at all.
I have seen the practice to start a database for running tests, but I feel it’s still very slow. And it makes sure that your tests assume you will always use SQL server as your data source, when the database is something related to the infrastructure and it is not part of the business logic.
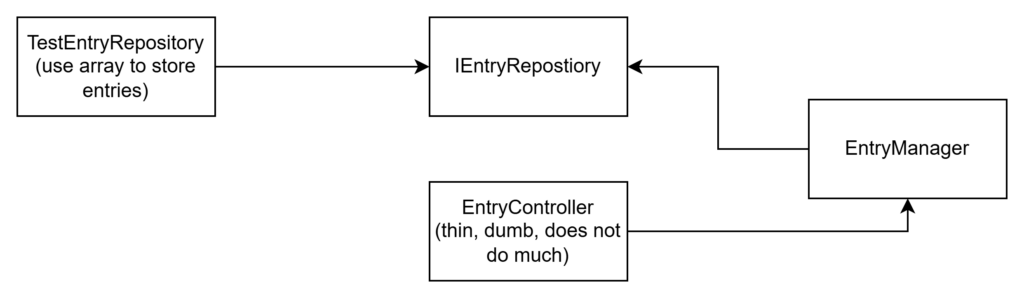
The benefits for designing this way: you can completely replace the backend data source with something else (MongoDB, Elasticsearch, another API). You can also add new API interface instead of just REST controllers, what about GraphQL?
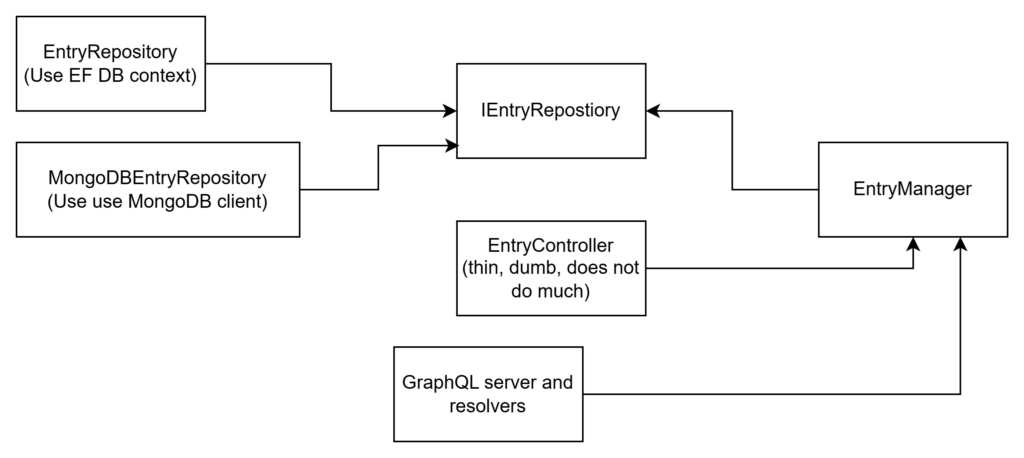
This only works if we implement so that
- The API interface is a very thin layer, the REST controllers (or GraphQL resolvers) do almost no business logic at all.
- The persistence layer (EntryRepository) should do no business logic aside from simple CRUD (Create, Read, Update, Delete).
- We put as much business logic as possible into the functional core (EntryManager) and cover it with tests.
In practice, I know that many projects rarely replace their database or API interface. But it’s fun nevertheless to try to implement this design.
With TDD, it’s pretty fun to work on, we almost never have to run the application manually to test its feature as the automated tests run very fast and can quickly verify that the software works. In many cases, I can make sure the tests pass, and the application runs correctly the first time I run it.
This is really helpful for a solo developer working on side projects like me, as the computer can do all the testing for me 😀 .
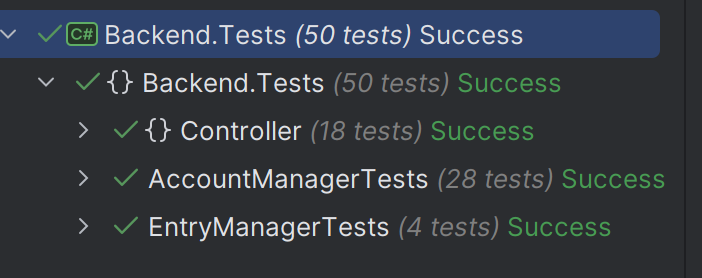
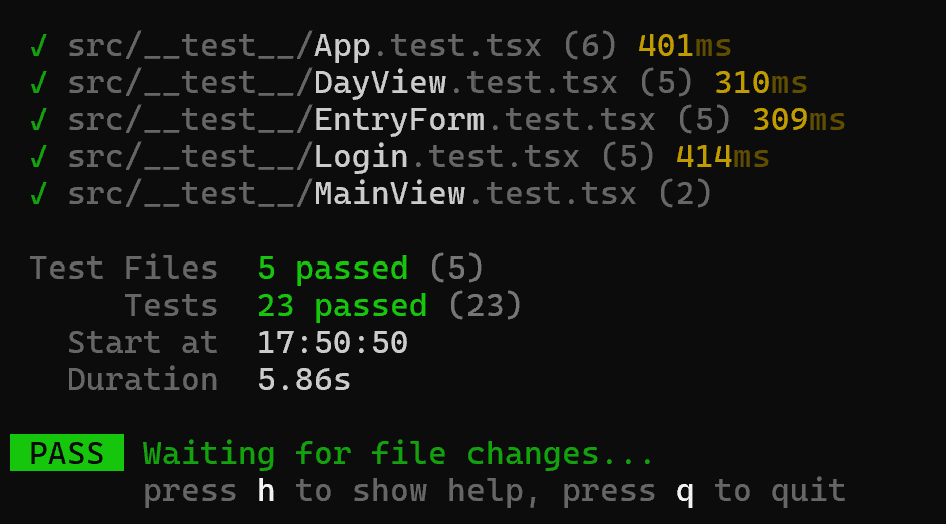